Hey there!
I’m in the process of downloading some images from an area of interest, and I need to keep them separate based on their TileID. You see, I have two different photos from the same date, and it’s crucial to distinguish between them. My initial approach was to save the files with just the date since I knew the next image wouldn’t be available for many days. However, it turns out that the subject area falls right on the border between two Sentinel tiles, complicating matters.
Here’s a snippet of the code I’m using:
aoi <- "C:/Users/1/Desktop/Work - BGU/juniper_RBD.gpkg"
from_date <- "2023-11-22"
to_date <- "2023-12-22"
library(openeo)
con <- connect(host = "https://openeo.dataspace.copernicus.eu")
openeo::login()
# Extracting bbox from the aoi file
catch = sf::st_read(aoi)
bbox_t = sf::st_bbox(obj = catch)
# get the process collection to use the predefined processes of the back-end
p = openeo::processes()
# get the collection list to get easier access to the collection ids, via auto completion
collections = openeo::list_collections()
# get the formats
formats = openeo::list_file_formats()
# load the initial data collection and limit the amount of data loaded
# note: for the collection id and later the format you can also use the its character value
cube = p$load_collection(id = collections$SENTINEL2_L2A,
spatial_extent = bbox_t,
temporal_extent = c(from_date, to_date),
bands = c('B01', 'B02', 'B03', 'B04', 'B05', 'B06', 'B07', 'B08', 'B8A', 'B09', 'B11', 'B12'),
properties = list(
"eo:cloud_cover" = function(x) x <= 10))
veg_index = "SAVI"
#' @title Calculate Vegetation Index function
calculate_vi_ <- function(x, context){
# loading bands colors
blue = x["B02"]
green = x["B03"]
red = x["B04"]
nir = x["B08"]
if (veg_index == "NDVI") {
vi_rast = ((nir - red) / (nir + red))
} else if (veg_index == "SAVI") {
vi_rast = ((1.5 * (nir - red)) / (nir + red + 0.5) )
} else if (veg_index == "MSAVI") {
vi_rast = ((2 * nir + 1 - sqrt((2 * nir + 1)^2 -
8 * (nir - red))) / 2)
} else if (veg_index == "CI") {
vi_rast = (1-((red - blue) / (red + blue)))
} else if (veg_index == "BSCI") {
vi_rast = ((1-(2*(red - green))) /
(terra::mean(green, red, nir, na.rm = TRUE)))
} else {
message("Unrecognized index: ", veg_index)
vi_rast = NULL
}
return(vi_rast)
}
cube_s2_vi = p$reduce_dimension(data = cube, reducer = calculate_vi_, dimension = "bands")
result = p$save_result(data = cube_s2_vi, format = formats$output$GTiff, options = list(filename_prefix=veg_index))
job_1 = openeo::create_job(graph = result, title = "check prefix")
openeo::start_job(job = job_1, log = TRUE)
jobs_vi = openeo::list_results(job = job_1)
openeo::download_results(job = job_1, folder = "C:/Users/1/Desktop/c2/check")
As you can see, the filenames of the saved files only include the date of the photo. However, since the subject area straddles two tiles, I need a way to differentiate between them. Any ideas on how I could achieve this?
My output:
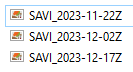
Expected:
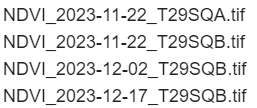
You can ignore the vi index; it’s just for the example
Looking forward to your suggestions!
Thanks,
Ron